Understanding Object-Oriented Programming (OOP) in Python: A Guide for High School Students
- Jashan Gill
- Jan 14
- 3 min read
If you’re learning Python and wondering what Object-Oriented Programming (OOP) is all about, you’re in the right place! OOP is a powerful programming paradigm that makes code more organized, reusable, and easier to understand. But what does that mean, and how can you relate it to your everyday life? Let’s break it down using real-life examples that are simple and fun.
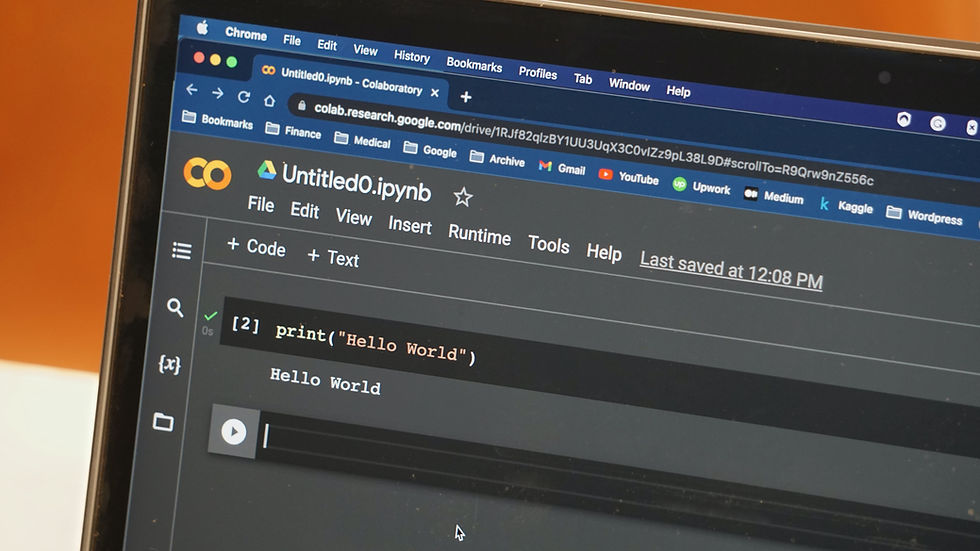
What is Object-Oriented Programming?
OOP is a way of organizing your code by creating "blueprints" for objects. Instead of writing repetitive code, you can create a general template (called a class) and then create specific instances (called objects) from that template.
Think of it like this: Imagine you’re designing a video game where there are different types of cars. Instead of coding each car individually, you create a Car class (the blueprint) and then generate specific cars (the objects) based on that blueprint.
Key Concepts of OOP
To understand OOP, let’s explore its four main principles using real-life examples.
1. Classes and Objects
Class: A class is like a blueprint or template.
Object: An object is a specific instance of a class.
Real-Life Example: Think of a class as a cookie cutter and objects as the cookies. The cookie cutter (class) defines the shape, but the actual cookies (objects) are the results.
In Python:
# Class definition
class Car:
def __init__(self, brand, color):
self.brand = brand
self.color = color
# Objects created from the class
car1 = Car("Tesla", "Red")
car2 = Car("Toyota", "Blue")
print(car1.brand) # Output: Tesla
print(car2.color) # Output: Blue
Here, Car is the class, and car1 and car2 are objects created from it.
2. Encapsulation
Definition: Encapsulation means bundling data and methods that operate on the data into one unit (class) and restricting direct access to some parts of the object.
Real-Life Example: Think of a washing machine. You only need to press a few buttons to make it work, but you don’t need to know how the internal mechanics operate. The machine encapsulates its inner workings.
In Python:
class BankAccount:
def __init__(self, owner, balance):
self.owner = owner
self.__balance = balance # Private variable
def deposit(self, amount):
self.__balance += amount
def get_balance(self):
return self.__balance
account = BankAccount("Alice", 1000)
account.deposit(500)
print(account.get_balance()) # Output: 1500
Here, __balance is a private variable that can only be accessed through methods like get_balance.
3. Inheritance
Definition: Inheritance allows one class to inherit attributes and methods from another class.
Real-Life Example: Think of a parent-child relationship. If your parent has blue eyes, you might inherit that trait. Similarly, a child class can inherit properties and methods from a parent class.
In Python:
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
return "I make a sound"
# Child class inheriting from Animal
class Dog(Animal):
def speak(self):
return "Woof!"
dog = Dog("Buddy")
print(dog.name) # Output: Buddy
print(dog.speak()) # Output: Woof!
Here, the Dog class inherits from the Animal class and customizes the speak method.
4. Polymorphism
Definition: Polymorphism means using a single interface to represent different types.
Real-Life Example:Think of the word “run.” A person can run, a program can run, and a clock can run. The action depends on the context, but the term remains the same.
In Python:
class Bird:
def fly(self):
return "I can fly high!"
class Penguin(Bird):
def fly(self):
return "I can't fly, but I swim well!"
bird = Bird()
penguin = Penguin()
print(bird.fly()) # Output: I can fly high!
print(penguin.fly()) # Output: I can't fly, but I swim well!
Both the Bird and Penguin classes have a fly method, but the behavior is different.
How to Practice OOP in Python
1. Start Small
Create classes for everyday objects, like a Book, Student, or Phone, and define their properties and methods.
2. Build a Mini Project
Try creating a simple project, such as:
A library management system where you can add, search, or issue books.
A car rental system with classes for cars and customers.
3. Use Online Resources
Practice on platforms like Codecademy, Khan Academy, or LeetCode to solve OOP problems.
Why Learn OOP?
Object-Oriented Programming isn’t just a skill; it’s a mindset. By mastering OOP in Python, you’ll:
Write cleaner, more organized code.
Build projects that mimic real-world scenarios.
Prepare yourself for advanced programming and software development.
Conclusion
Object-Oriented Programming may sound complex at first, but with practice and real-life examples, it becomes intuitive. Classes, objects, encapsulation, inheritance, and polymorphism are the building blocks of this approach, and Python makes it incredibly easy to implement them.
So, grab your laptop and start coding! The journey to mastering OOP starts with a single class. 🎓🐍✨
Want more tips and tutorials like this? Subscribe to our newsletter for step-by-step guides and fun coding challenges tailored for high school students! Click here to subscribe!
Comentários